18. Identifiers and Keyword in C programming
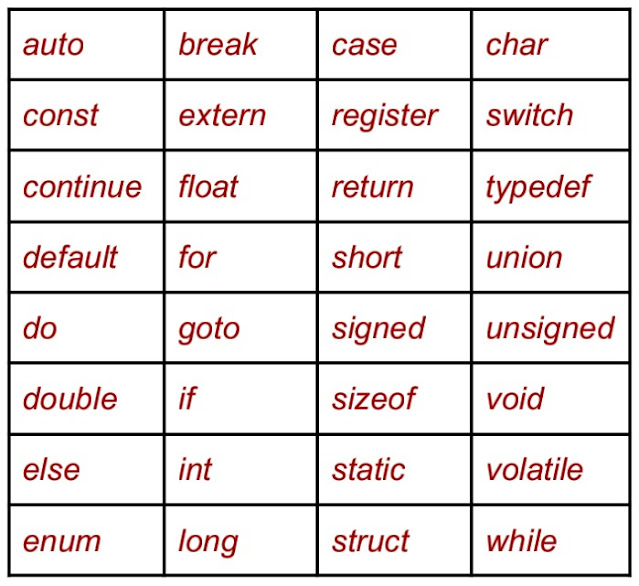
Identifiers & Keywords Identifier is the fancy term used to mean 'name'. In C, identifiers are used to refer to a number of things: we've already seen them used to name variables and functions. They are also used to give names to some things we haven't seen yet, amongst which are labels and the ‛tags’ of structures, unions, and enums. Keywords are the special terms reserved for a special purpose in a language, which cannot be used as variable names. Keywords are identifiers reserved by the language for special use. Every keyword has a special meaning. All keywords must be written in lower case letter. C language uses 32 keywords which are not available to users to use them as variables or function names. So keywords are the words whose meaning has already been explained to the C compiler. All the keywords are given below: Keywords in C Language